- Published on
leetCode 22.Generate Parentheses
- Authors
- Name
- Keira M J
leetCode 22. Generate Parentheses.
Problem
Given n
pairs of parentheses, write a function to generate all combinations of well-formed parentheses.
Example 1:
Input: n = 3
Output: ['((()))', '(()())', '(())()', '()(())', '()()()']
Example 2:
Input: n = 1
Output: ['()']
Constraints:
1 <= n <= 8
Approach
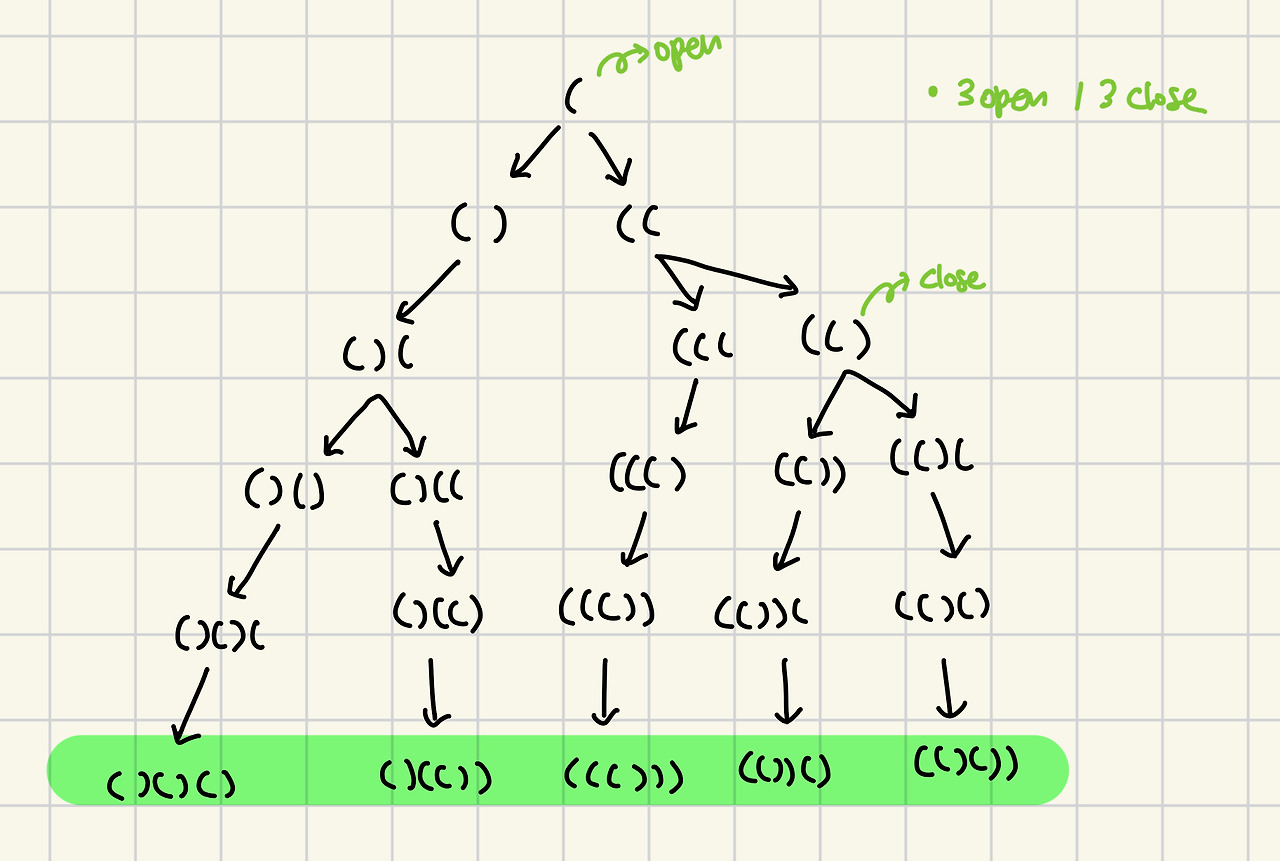
"("
, ")"
called "open" and "close" each other. I need 3 open, 3close. open must be first. close can not be first. always behind open. so that means 1 open , 0 close, or 1 open, 1close -> open > close
Solution
var generateParenthesis = function (n) {
const res = []
backtracking(0, 0, '')
return res
function backtracking(open, close, cur) {
if (close > open || open > n) return
if (open == n && close == n) {
res.push(cur)
return
}
backtracking(open + 1, close, cur + '(')
backtracking(open, close + 1, cur + ')')
}
}